Swift is a powerful and intuitive programming language developed by Apple for iOS, macOS, watchOS, and tvOS app development. Below is a basic tutorial to get you started with Swift:
In Swift, you can declare variables using the var
keyword and constantsf constants using the let
keyword. For example:
var myVariable = 42
let myConstant = 3.14159
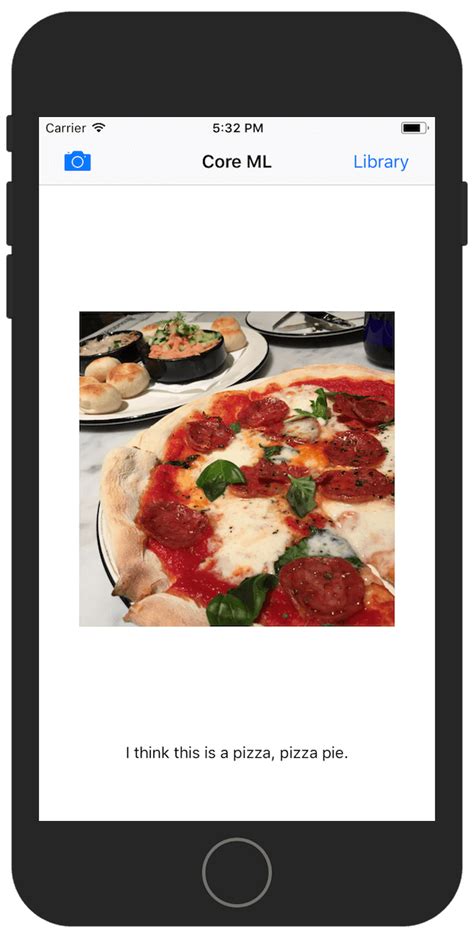
Swift supports various data types such as integers, floatingpoint numbers, strings, arrays, dictionaries, and more. It also supports type inference, allowing you to write cleaner code without explicitly specifying the data type.
Swift provides traditional control flow mechanisms like if
, for
, while
loops, as well as powerful features like switch
statements with support for pattern matching and guard
statements for early exits.
Functions in Swift are firstclass citizens, meaning you can define functions inside other functions, pass functions as arguments to other functions, and return functions from functions. Swift also supports closures, which are selfcontained blocks of functionality that can be passed around and used in your code.
Optionals are a key feature of Swift that allows you to represent both a value and the absence of a value. This helps in handling situations where a variable may or may not have a value. You can use optional binding or optional chaining to safely work with optionals.
Swift supports both classes and structures for defining custom data types. Classes support inheritance and reference counting, while structures are value types. You can also define properties, methods, and subscripts in your classes and structures.
Swift provides the trycatch
mechanism for handling errors. You can use dotrycatch
blocks to catch and manage errors thrown by functions that can potentially fail. Swift also supports throwing custom errors using enums.
Swift uses Automatic Reference Counting (ARC) to manage memory. ARC keeps track of instances of classes to know whether they are still in use, and automatically deallocates instances when they are no longer needed.
In Swift, you can use Swift Package Manager to manage dependencies and thirdparty libraries in your projects. This makes it easy to integrate external libraries and frameworks into your Swift projects.
To further your understanding of Swift, you can refer to the following resources:
With these resources and the basics covered in this tutorial, you can start your journey into app development using Swift!