```python
在编程中,判断一个年份是否为闰年是一项常见的任务。闰年是指能被4整除但不能被100整除,或者能被400整除的年份。下面是几种常见编程语言的实现方法:
def is_leap_year(year):
if (year % 4 == 0 and year % 100 != 0) or (year % 400 == 0):
return True
else:
return False
year = 2024
if is_leap_year(year):
print(f"{year}是闰年")
else:
print(f"{year}不是闰年")
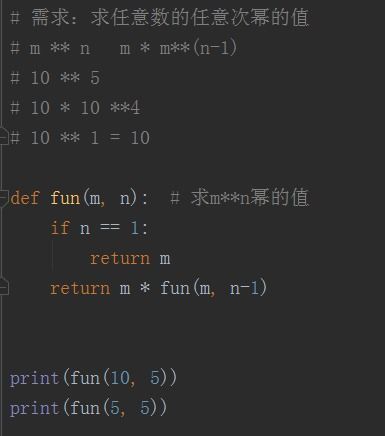
function isLeapYear(year) {
return (year % 4 === 0 && year % 100 !== 0) || (year % 400 === 0);
}
const year = 2024;
if (isLeapYear(year)) {
console.log(`${year}是闰年`);
} else {
console.log(`${year}不是闰年`);
}
include <iostream>
bool isLeapYear(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
int main() {
int year = 2024;
if (isLeapYear(year)) {
std::cout << year << "是闰年" << std::endl;
} else {
std::cout << year << "不是闰年" << std::endl;
}
return 0;
}
以上是几种常见编程语言的实现方法。你可以根据自己的需求选择其中一种来判断一个年份是否为闰年。